Documentation
Introduction
Welcome to our documentation. Here, you'll find all the information you need to get started with our Database as a Service (DaaS) boilerplate.
Our DaaS boilerplate is a full-stack application that uses Next.js, Chakra UI, PostgreSQL, and Strapi (Node.js). We've designed it to simplify the process of selling your database.
Next.js is a powerful React framework that offers features like Server-Side Rendering (SSR), Static Site Generation (SSG), and Incremental Static Regeneration (ISR). We've chosen to use the Pages router for its stability and extensive community support. Based on our experience, the App Router is not yet fully ready for all production use cases.
We've chosen Chakra UI for its intuitive API and clean syntax. Chakra UI provides a more straightforward way of writing CSS, making it easier to create and maintain components. Here's an example of how we've built our FAQ component:
We use PostgreSQL as our relational database. You won't need to write raw SQL queries, as Strapi handles that for you:
Strapi is a leading open-source Content Management System (CMS). You only need to define your data structure, and Strapi takes care of the rest, including API generation, content management, role-based access control, and more.
A key advantage of this boilerplate is its use of Docker, which simplifies the development and deployment process. Docker eliminates the need to manually install and configure multiple dependencies like PostgreSQL, Node.js, Yarn, and npm. It ensures consistency across different environments, making it easier to move from development to production.
Setup
To get started, you'll need Visual Studio Code (VSCode) with the Docker extension installed. If you see a "Docker Daemon not running" message, don't worry - we'll address that in the following steps. Let's begin with the Docker installation:
1. Installing Docker
Docker is essential for our setup process. Here's how to install it on your computer:
- Go to the official Docker website and download Docker Desktop for your operating system (Mac, Windows, or Linux).
- Follow the installation instructions provided on the Docker website.
- For Windows users:
- We recommend using the Hyper-V version. You can find the installation guide here: Docker Desktop for Windows
- If you need to enable Hyper-V, follow this guide: Enabling Hyper-V
- After enabling Hyper-V, restart your computer.
- Once installed, run Docker Desktop.
- Open Visual Studio Code and click on the Docker extension. It should now show that Docker is ready to use.
Note: For production server deployment, the installation process is simpler and requires just a few terminal commands.
If you encounter any issues during the installation process, please don't hesitate to reach out to us in our Discord server. We're here to help!
2. Clone the Repository
Clone the repository to your computer after accepting the GitHub invitation. If you haven't received an invitation, check your email for instructions sent after your purchase.
On Windows, we recommend using PowerShell (as administrator) instead of the standard Command Prompt to avoid potential issues.
3. Install NVM (Node Version Manager)
We use NVM to manage Node.js versions. This allows you to have multiple versions of Node installed and easily switch between them.
- Visit this guide to install NVM.
- Open a terminal (PowerShell / Command Prompt) and navigate to the cloned repository folder. Type
code .
and press Enter to open VSCode in that folder. - Open a terminal in VSCode. Choose a profile that has NVM's PATH set correctly. Test it by typing
nvm -v
. If it doesn't work, revisit the NVM installation guide to ensure the PATH is set correctly. - In the terminal, run
nvm install v18.19.1
to install the correct Node.js version. - Then, run
nvm use v18.19.1
to use this version. - Verify the installation by running
node -v
. NPM should also be installed. - Install Yarn globally by running
npm install -g yarn
. Verify the installation withyarn -v
.
You have now successfully installed all necessary tools. Proceed to the next section to continue.
Configuration
Before running the project, we need to make some code edits. Open the project in VSCode and follow these steps:
Locate the three .env.example files by pressing CMD+P (or Ctrl+P on Windows) and typing "example".
Rename each file by removing the ".example" extension, making them proper .env files.
Fill in the correct information in each .env file.
Note: There's an .env file in each folder (Root, Backend, and Frontend). Each folder will become a Docker container with its own environment variables.
We use Traefik as a reverse proxy instead of NGINX/APACHE to direct your domain name to your project. You can see this configuration in the docker-compose.yml file. Traefik is currently commented out as it's not needed for local development.
Installing Node Modules
Before running the project, install the node modules. Follow these steps in your VSCode terminal:
Navigate to the backend folder:
cd backend
Install backend dependencies:
yarn install
Navigate to the custom plugin directory:
cd src/plugins
Install custom plugin dependencies:
yarn install
Build the custom plugin:
yarn build
Return to the root directory:
cd ../../..
Navigate to the frontend folder:
cd frontend
Install frontend dependencies:
yarn install
Running the Project
To start the project:
In the VSCode terminal, navigate to the project root.
Run the command:
docker compose up
This process may take some time as it downloads and installs all necessary components.
Once complete, you'll see localhost:3005 and localhost:1337/admin running in the terminal logs.
localhost:1337 is your backend/Strapi admin panel. Visit localhost:1337/admin to register with an email and password (for local use only).
localhost:3005 is your NextJS frontend.
Backend Admin Panel Configuration
After setting up your project, you need to configure some settings in the backend admin panel:
Go to the Strapi admin panel at localhost:1337/admin.
Navigate to the Webhook section and set the name field as 'webhook'.
In the Config section, choose a template for your site.
Set the 'seed_database' option to true. This will fill your database with dummy data that you can later edit.
Remember to save your changes.
Stripe Configuration
To set up Stripe for payments:
Create a Stripe account if you haven't already.
In your Stripe dashboard, navigate to the Developers section.
Go to Webhooks and click "Add endpoint".
Set the webhook URL to:
https://api.{projectname}.com/api/webhook/webhook
Select the events you want to listen for (typically 'checkout.session.completed').
Save the webhook configuration.
Strapi Configuration
The initial configuration is already set up in the .env files. The remaining setup is done in the Strapi admin panel. Take some time to explore the admin panel, but avoid making changes for now.
Key concepts to understand:
Content-Type Builder: Define the structure of your data
Content Manager: Add and edit your actual data
API endpoints: Automatically generated for each data type
We've already set up many features for you:
Database seeded with sample data
Necessary API endpoints enabled
Structures for FAQs, Products, Testimonials, and Blogs
To add or edit data, go to the Content Manager, select a collection type, and make your changes. For Testimonials and Blog posts, you can upload images directly in their respective entries.
This project uses a data fetching method called SWR, which provides real-time updates similar to websockets. When you change data in the backend, it automatically updates on the frontend without needing to reload the page. You can test this by editing something like a product title in the backend, then switching back to the frontend to see the change immediately. In the Content Manager, you'll find a Config button. This opens a menu where you can choose from several pre-made homepage templates. These templates are designed to improve conversion rates (proven), and you can select the one that best fits your needs.
Email Configuration
We use PostmarkApp for sending emails. It's user-friendly and offers $75 in startup credit for new SaaS companies. To set it up:
Register with PostmarkApp
Complete their verification process
Explain your use case (remember, only transactional emails are allowed)
Blogs
Our platform provides a user-friendly way to create and manage blog posts directly from the Strapi admin backend panel.
The blog editor in Strapi features a WYSIWYG (What You See Is What You Get) interface, making it easy to format your content without needing to know HTML or markdown.
When creating a blog post, you can add images directly in the content section. It's important to note that the first image you add to the content will automatically be used as the header image for your blog post.
This streamlined approach allows you to focus on writing great content while the system takes care of the visual presentation of your blog posts.
Import Database
The next crucial step is importing the database you want to provide to your customers.
Ensure your database is in CSV format.
Open your CSV file with a text editor and note the column names.
Example of column names: First Name, Last Name, Full Name, Company, Website, Email, Phone
Important: Remove any trailing spaces from the column names within the csv itself.
Next, set up your database structure in the admin panel:
In the sidebar, click on 'Database Sync'. You'll see a text box where you can paste the content of your .csv file. After pasting, click 'Sync Data' to start importing.
This will import your database into your project. Keep in mind that larger databases will take more time to import.
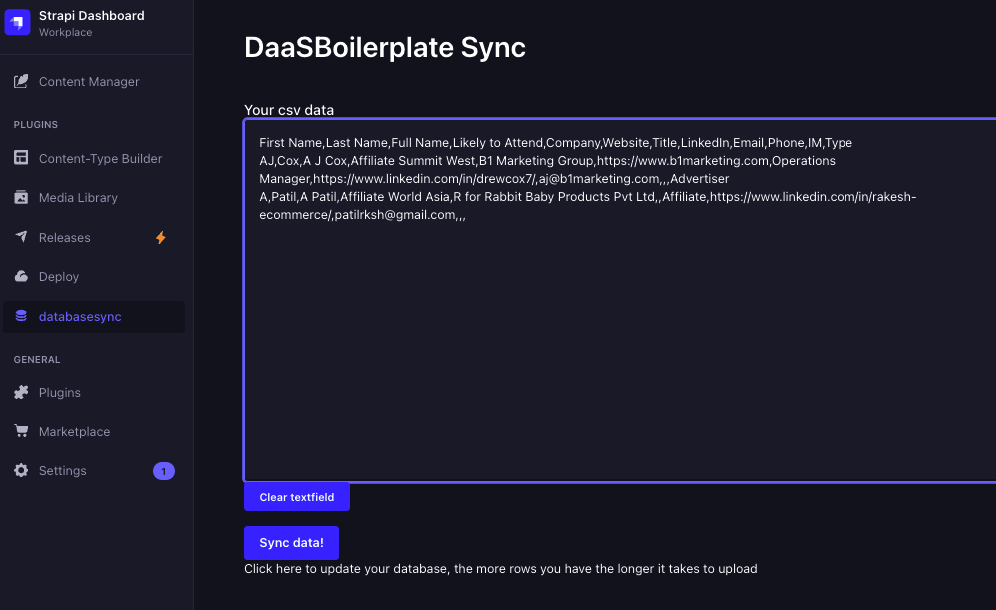
After importing, users can make 3 free searches in the /free section of your website. When a user buys one of your products and signs up, they'll get an email with a login link. After logging in, they can access the full /database section.
Our system automatically creates filter options in the /database sidebar based on your database content. This makes it easier for users to search and find information.
Important Note:
We used this system to create submitSites.com. When you signed up, you got an email to access this GitHub repo. That specific code is commented out in the /controllers/webhook.ts file. Instead, we've added code for the login link.
Theming
1. We use Chakra UI as our CSS library. Please refer to their documentation for available components: Chakra UI Components
2. To edit your theme colors, go to the "theming.ts" file in your project. We used coolors.co to generate our brand colors: